What is DOT Sourcing?
So what exactly is the DOT source method and what does it do? Well have you ever created a bunch of functions inside a script run the script and then noticed after the script runs you can’t use any of the functions you previously wrote? This is because the functions are temporarily loaded in memory within the scope of the script. However, DOT sourcing allows us to load functions into the GLOBAL scope. These can then be accessed by scripts, the PowerShell console or really by anything for the duration of the PowerShell session. DOT Sourcing is an easy way to create a module without extra work.
The DOT Source method while similar to running the “Import-Module” command is different. When we use the “import-module” command we import an entire manifest of information and sometimes compiled code. Most infrastructure admins can safely say we don’t write compiled code.
The entire purpose of using the DOT source method is essentially to learn how to be lazy. It allows us an easy way to re-use code we have already written without a bunch of extra work.
How and when should we use it?
Let’s jump into some real-world examples. Maybe you’re an admin, and you have a folder full of scripts and periodically you open file explorer, and run a particular script. Maybe you are writing a new script and want to use a logging function you wrote three weeks ago to explain what this script does. Instead of copying and pasting a file or writing an entire module you could simple use the DOT source method to make those other functions available. Let’s see what this looks like, first we need a “script” this script won’t actually do anything it just has a few previously defined functions in it. You can copy the below code block or download it from the TrueSec public GitHub and save it as a PS1. The code can be downloaded below:
function write-GreenMessage { [cmdletbinding()] param( [parameter(Mandatory=$false)] [string]$Message ) $originState = $Host.UI.RawUI.ForegroundColor $Host.UI.RawUI.ForegroundColor = "Green" Write-Output -inputobject $Message $Host.UI.RawUI.ForegroundColor = $originState } function write-YellowMessage { [cmdletbinding()] param( [parameter(Mandatory=$false)] [string]$Message ) $originState = $Host.UI.RawUI.ForegroundColor $Host.UI.RawUI.ForegroundColor = "Yellow" Write-Output -inputobject $Message $Host.UI.RawUI.ForegroundColor = $originState } function write-RedMessage { [cmdletbinding()] param( [parameter(Mandatory=$false)] [string]$Message ) $originState = $Host.UI.RawUI.ForegroundColor $Host.UI.RawUI.ForegroundColor = "Red" Write-Output -inputobject $Message $Host.UI.RawUI.ForegroundColor = $originState }
Above is a simple function. This function writes a success message back in Green, Yellow or Red. I have saved this as “Write-Functions.PS1” in my C:Scripts folder as an example. Our next step is to open a PowerShell prompt and since we are writing messages no need to run it as an administrator. Next I will navigate to the C:Scripts directory and import my functions.
set-location -path C:Scripts #Now we use Dot Sourcing to import all the functions into global memory. . .Write-Functions.Ps1
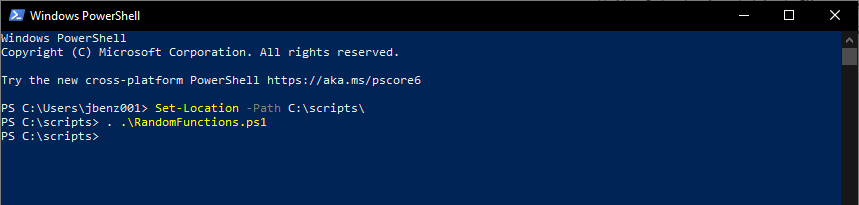
Once we’ve done this all of the functions stored in the .PS1 file will be available as commands we can run from PowerShell! Let’s test it out.
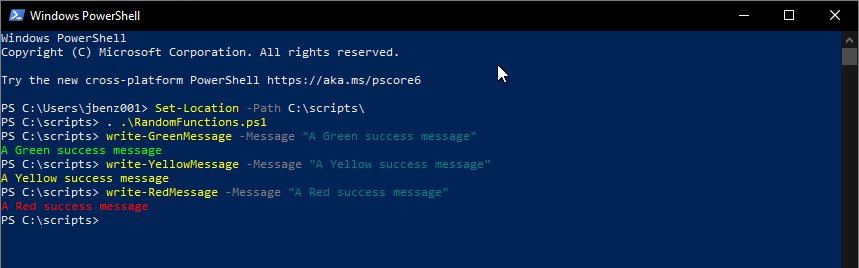
What about lots of files with just one function in them?
What makes this method so cool is there is no requirement to stick all of the functions in one file. You could just create one function per file and then run a simple PowerShell loop command to load all of the functions into memory. Here is an example assuming we broke out our above functions into three files.
Get-ChildItem -Path "C:Scripts" | ForEach-Object {. $($_.FullName)}
This would import the functions of every script in the scripts folder into memory until the window was closed.
Conclusion and till next time
While PowerShell DOT sourcing isn’t talked about often it can be a real time saver if you write a lot of small functions. The sticking point is why would you use this over a module, and the answer is because we are lazy. Not every script or function ever written to be placed in a module.
I hope this was a helpful guide on how and why you might want to use DOT Sourcing in PowerShell. The goal, after all, is always to write re-usable code.
Want to learn more?
If you need an even deeper understanding and training join Geek Week in March. A great time to ask him more questions about this or other findings and take your skills to the next level!
Jordan Benzing is one of the instructors on Geek Week and will be holding the class “PowerShell v5+ training – Use PowerShell every day!”